Learn JavaScript methods in Marathi with examples. These array methods are asked in frontend developers interview (JavaScript Array Methods with example).
1) at(): method हि indexed element ला array मधून return करते.
Example:
<script>
function checkatMethod(){
const herosList = ["Captain America", "Thanos", "Spiderman", "Batman"];
let hero = herosList.at(2);
console.log(hero);
}
checkatMethod();
</script>
Output:
Spiderman
2) concat(): दोन किंवा अधिक array हे join किंवा concat करण्यासाठी concat() हि method वापरली जाते.
concat() method हि नवीन array ला रिटर्न करते तसेच existing array ला change करत नाही.
Example:
<script>
const arr1 = ["Spiderman", "Iornman"];
const arr2 = ["Marvel", "Studios", "Antman"];
const children = arr1.concat(arr2);
console.log(children);
</script>
output:
Spiderman,Iornman,Marvel,Studios,Antman
3) entries(): method हि array मधून Iterator object ला key/value pairs मध्ये return करते. entries() method हि original array ला change करत नाही.
Example
<script>
// Create an Array
const basket = ["Banana", "Orange", "Apple", "Mango"];
// Create an Iterator
const fruitList = basket.entries();
// List the Entries
let text = "";
for (let x of fruitList) {
text += x + "<br>";
}
console.log(text);
</script>
output:
0,Banana
1,Orange
2,Apple
3,Mango
4) every(): array मधील प्रत्येक element साठी every() method हि function ला execute करते. जर function array मधील सर्व elements साठी true return करत असेल तर every() देखील true रिटर्न करते, परंतु जर function जर array elements मधील एक value जरी false return करत असेल तर every() method हि false value रिटर्न करते.
every() method empty elements साठी function return करत नाही तसेच original array ला देखील change करत नाही.
Example
<script>
// Create an Array
const ages = [32, 33, 16, 40];
// Function to Run for every Element
function checkAge(age) {
return age > 18;
}
console.log("Is every element over 18? " + ages.every(checkAge));
</script>
output:
Is every element over 18? false
5) fill(): fill() method वापरून आपण array मध्ये specified elements fill करू शकतो. fill() method original array ला overwrite करते. start आणि end position आपण specify करू शकतो जर नाही केली तर सर्व elements हे fill होऊन जातील.
Example:
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
const result = fruits.fill("Kiwi");
console.log(result);
</script>
output:
Kiwi,Kiwi,Kiwi,Kiwi
6) filter(): method जर function मधील condition satisfy झाली तर array elements चा नवीन array create करते. original array ला change करत नाही.
<script>
const ages = [32, 33, 16, 40];
console.log(ages.filter(checkAdult));
function checkAdult(age) {
return age >= 18;
}
</script>
output:
32,33,40
7) find(): method हि function मधील condition नुसार array मध्ये match झालेले first element ला return करते. find() method array मधील प्रत्येक element साठी function ला execute करते. जर condition नुसार array element नाही सापडला तर undefined return करते.
find() method हे empty elements साठी function execute करत नाही तसेच original array ला देखील change करत नाही.
Example:
<script>
const ages = [3, 10, 18, 20];
const result = ages.find(checkAge);
console.log(result);
function checkAge(age) {
return age > 18;
}
</script>
output:
20
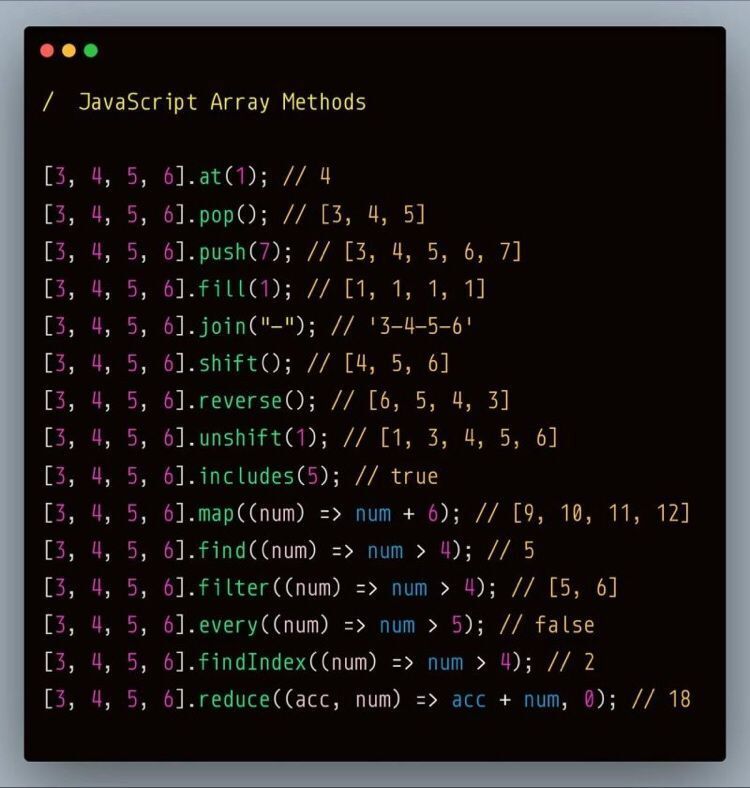
8) flat(): method array मध्ये असणाऱ्या sub-array elements ला concat करते.
Example:
<script>
const myArr = [[1,2],[3,4],[5,6]];
const newArr = myArr.flat();
console.log(newArr);
</script>
output:
1,2,3,4,5,6
9) forEach(): method array मधील प्रत्येक elements साठी function ला कॉल करते.
Example:
<script>
let text = "";
const fruits = ["apple", "orange", "cherry"];
fruits.forEach(myFunction);
console.log(text)
function myFunction(item, index) {
text += index + ": " + item + "<br>";
}
</script>
output:
0: apple
1: orange
2: cherry
10) from(): method हि कोणत्याही iterable object किंवा string पासून array return करते ज्याला length प्रॉपर्टी देखील असते.
Example:
<script>
let text = "ABCDEFG"
const myArr = Array.from(text);
console.log(myArr);
</script>
output:
A,B,C,D,E,F,G
11) includes(): method जर array मध्ये specified value असेल तर true return करते नाहीतर false return करते.
Example:
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
console.log(fruits.includes("Mango"));
</script>
output:
true
12)indexOf(): method हि specified value साठी first index (position) ला return करते. जर specified value सापडली नाही तर -१ return होईल. indexOf() specified index पासून start होते आणि left (start) पासून right(end) ह्या pattern ने search केले जाते.
जर start value negative असेल तर value count last element पासून सुरु होतो.
Example:
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
let index = fruits.indexOf("Apple");
console.log(index);
</script>
Output:
2
1 thought on “JavaScript Array Methods with example | Learn in easy language | most frequently asked array related questions in interview 2024 | JavaScript methods in Marathi (Part 1)”