In this blog we are going to discus about predefined JavaScript methods which we are going to use in our Industrial Projects. Here we are providing javascript array methods list.
javascript array methods list:
1) isArray(): जर object हा array असेल तर isArray() method true return करेल नसेल तर false.
Example:
<script>
const orgs = ["Big", "Ola", "Amazon", "Meta"];
let result = Array.isArray(orgs);
console.log(result);
</script>
Output:
true
2) join(): join method array ला as a String म्हणून रिटर्न करते. हि method original array ला change करत नाही. तसेच आपण syntax मध्ये कोणताही separator वापरू शकतो. Default separator हा comma (,) आहे.
Example:
<script>
const orgs = ["Big", "Ola", "Amazon", "Meta"];
let text = orgs.join(" and ");
console.log(text);
</script>
Output:
Big and Ola and Amazon and Meta
3) keys(): method iterator object ला array च्या keys सहित return करतो. तसेच ओरिजिनल array ला change करत नाही.
Example:
<script>
// Create an Array
const orgs = ["Big", "Ola", "Amazon", "Meta"];
// Create an Iterable
const list = orgs.keys();
// List the Keys
let text = "";
for (let x of list) {
text += x + "<br>";
}
console.log(text);
</script>
Output:
0
1
2
3
4)length(): method हि array मध्ये किती elements आहेत ते return करते तसेच length सेट करण्यासाठी देखील वापरली जाऊ शकते.
Example:
<script>
const orgs = ["Big", "Ola", "Amazon", "Meta"];
let length = orgs.length;
console.log(length);
</script>
Output:
4
5) map(): method() array मधील प्रत्येक element साठी function call करून नवीन array तयार करतो. हि method original array ला change करत नाही.
Example:
<script>
const numbers = [65, 44, 12, 4];
const newArr = numbers.map(myFunction);
console.log(newArr);
function myFunction(num) {
return num * 10;
}
</script>
output:
650,440,120,40
6) pop(): method हि array मधील शेवटचे element (last element) ला remove करते. हि method original array ला change करते आणि remove केलेल्या element ला return करते.
Example:
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
let removed = fruits.pop();
console.log(removed);
</script>
Output:
Mango
7) prototype(): method आपल्याला array मध्ये नवीन property किंवा method add करण्यास मदत करते.
Example:
<script>
// Add a new method
Array.prototype.myUcase = function() {
for (let i = 0; i < this.length; i++) {
this[i] = this[i].toUpperCase();
}
};
// Use the method on any array
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.myUcase();
console.log(fruits);
</script>
Output:
BANANA,ORANGE,APPLE,MANGO
8) push(): method हि array च्या शेवटी नवीन item add करण्यासाठी वापरला जातो. हि method array ची length change करते व नवीन array return करते.
Example:
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.push("Kiwi", "Lemon");
console.log(fruits);
</script>
output:
Banana,Orange,Apple,Mango,Kiwi,Lemon
9) reverse(): method array मधील असणाऱ्या elements ची order reverse करते आणि original array ला overwrite करते.
Example:
<script>
// Create an Array
const fruits = ["Banana", "Orange", "Apple", "Mango"];
// Reverse the Array
const fruits2 = fruits.reverse();
console.log(fruits2);
</script>
output:
Mango,Apple,Orange,Banana
10) shift(): method array मधील first item ला remove करते त्यामुळे original array देखील change होतो आणि हि method shift केलेल्या item ला रिटर्न करते.
Example:
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
console.log(fruits.shift());
</script>
Output:
Banana
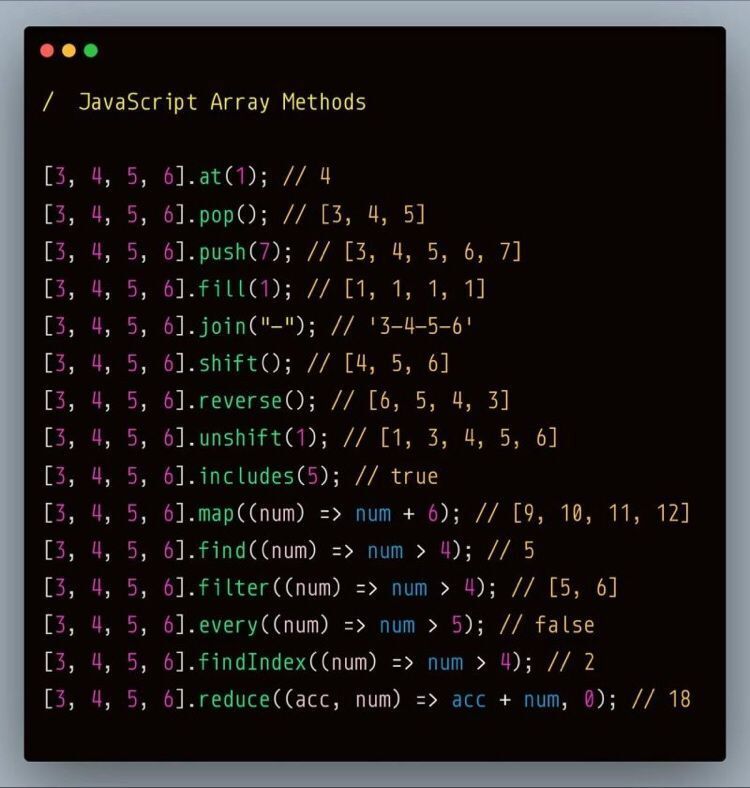
11) slice(): method जे element select केले आहे त्यांना as a new array म्हणून return करते. slice() method original array ला change करत नाही.
Example:
<script>
const fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"];
const citrus = fruits.slice(1, 3);
console.log(citrus);
</script>
Output:
Orange,Lemon
12) sort(): method array elements ना sort करण्यासाठी वापरली जाते. sort method हि elements ला as string in alphabetical आणि ascending order ने सॉर्ट करते. हि method original array ला overwrite करते.
Example:
<script>
// Create an Array
const fruits = ["Banana", "Orange", "Apple", "Mango"];
// Sort the Array
fruits.sort();
// Display the Array
console.log(fruits);
</script>
output:
Apple,Banana,Mango,Orange
13) splice(): method array elements ला add किंवा remove करते आणि original array ला overwrite करते.
Example:
<script>
// Create an Array
const fruits = ["Banana", "Orange", "Apple", "Mango"];
// At position 2, add "Lemon" and "Kiwi"
fruits.splice(2, 0, "Lemon", "Kiwi");
console.log(fruits);
</script>
Output:
Banana,Orange,Lemon,Kiwi,Apple,Mango
14) toString(): method array मध्ये असलेल्या comma separated values ला string म्हणून return करते.
Example:
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
let text = fruits.toString();
console.log(text);
</script>
Output:
Banana,Orange,Apple,Mango
15) unshift(): method array चा सुरवातीला नवीन element ला add करते.
Example:
<script>
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.unshift("Lemon", "Pineapple");
console.log(fruits);
</script>
Output:
Lemon,Pineapple,Banana,Orange,Apple,Mango
16) values(): method array च्या value असलेले Iterator object ला return करते.
Example:
<script>
// Create an Array
const fruits = ["Banana", "Orange", "Apple", "Mango"];
// Create an Iterator
const list = fruits.values();
// List the Keys
let text = "";
for (let x of list) {
text += x + "<br>";
}
console.log(text);
</script>
Output:
Banana
Orange
Apple
Mango
17) with(): method specified array element ला update करते व नवीन array ला रिटर्न करते.
Example:
<script>
const months = ["Januar", "Februar", "Mar", "April"];
const myMonths = months.with(2, "March");
console.log(myMonths);
</script>
Output:
Januar,Februar,March,April