1) Explain template-driven forms and reactive forms in Angular (angular forms interview questions in marathi).
Angular मध्ये template-driven forms आणि reactive forms हे user चे form मधील input manage करण्यासाठीचे mechanism आहे.
Template-driven Forms:
- Template-driven forms हे angular template syntax वापरून तयार केले जाते. इथे form controls हे directly HTML template मध्ये define केलेले आहेत.
- form validation हे required, minLength, maxLength, etc सारख्या angular directives वापरुन directly html template मध्ये केले जाते
- Template-driven forms हे लहान किंवा simpler forms साठी basic validation सहित चांगला पर्याय आहे
- Template-driven forms मध्ये data handling आणि validation logic हे template मधेच manage केले जाते
- Template-driven forms हे छोट्या लेव्हलच्या ऍप्लिकेशनसाठी किंवा असे फॉर्म ज्यामध्ये validation हे straightforward असेल अशा साठी अधिक चांगला पर्याय आहे. परंतु जेव्हा form हा अधिक complex आणि validation पण specific field नुसार असतात त्यावेळेस आपल्याला reactive forms हा पर्याय योग्य ठरतो.
Example:
<form #myForm="ngForm">
<input type="text" name="name" ngModel required>
<button type="submit" [disabled]="!myForm.valid">Submit</button>
</form>
Reactive Forms:
- reactive forms हे Angular चे FormBuilder service आणि FormGroup आणि FormControl classes हे वापरून programmatically built केले जाते.
- Form controls हे component class मध्ये define केले जाते ज्यामुळे form चा चांगला control आणि flexibility मिळते.
- validation logic हे component class मध्ये built-in validators किंवा custom validator function चा वापर करून लिहले जाते
- Reactive forms हे complex forms आणि dynamic validation तसेच काही advanced scenarios handle करण्यासाठी चांगला पर्याय आहे.
Example: ts file
import { Component, OnInit } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
@Component({
selector: 'app-my-form',
templateUrl: './my-form.component.html',
styleUrls: ['./my-form.component.css']
})
export class MyFormComponent implements OnInit {
myForm: FormGroup;
constructor(private fb: FormBuilder) { }
ngOnInit() {
this.myForm = this.fb.group({
name: ['', [Validators.required, Validators.minLength(3)]],
email: ['', [Validators.required, Validators.email]],
password: ['', [Validators.required, Validators.minLength(6)]]
});
}
onSubmit() {
if (this.myForm.valid) {
// Form submission logic
}
}
}
html template:
<form [formGroup]="myForm" (ngSubmit)="onSubmit()">
<input type="text" formControlName="name">
<input type="email" formControlName="email">
<input type="password" formControlName="password">
<button type="submit" [disabled]="myForm.invalid">Submit</button>
</form>
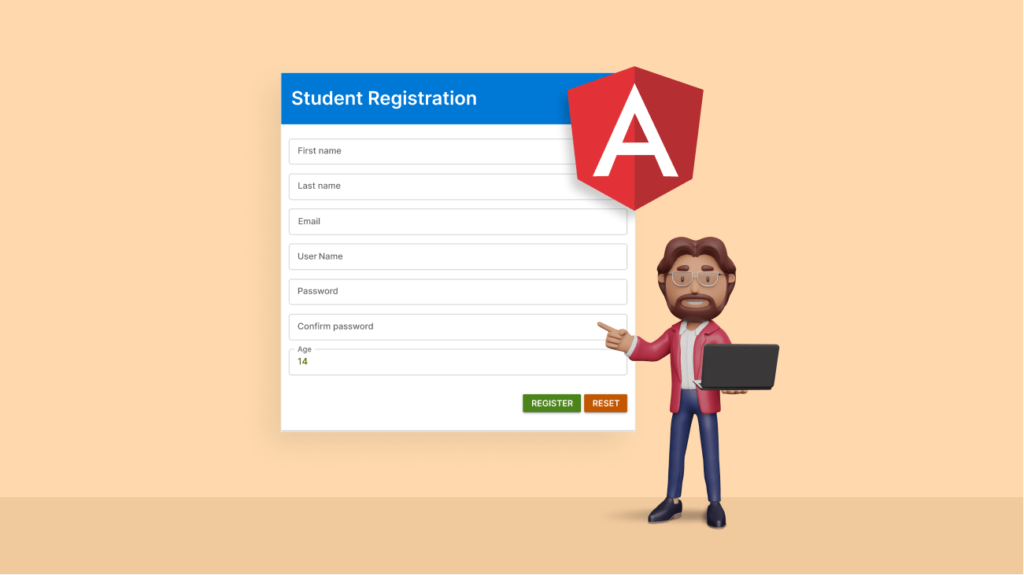
2) How do you perform form validation in Angular for reactive form? (angular forms interview questions in marathi)
Reactive form मध्ये validations add करण्यासाठी आपल्याला खालील steps follow कराव्या लागतील
1) Create the Form
2) Bind to Template
3) Display Validation Errors
4) Custom Validators
5) Handling Form Submission
आता आपण वरील steps follow करून example बघूया
Create the Form: आपल्या component / typescript file मध्ये form control आणि group हे FormGroup च्या आत define करून घ्या.
example:
import { FormGroup, FormControl, Validators } from '@angular/forms';
export class MyComponent {
myForm: FormGroup;
constructor() {
this.myForm = new FormGroup({
name: new FormControl('', Validators.required),
email: new FormControl('', [Validators.required, Validators.email]),
// add more form controls as needed
});
}
}
Bind to Template: आता आपल्या HTML Template फाईल मध्ये form controls हे formControlName directive वापरून bind करून घ्या.
example:
<form [formGroup]="myForm" (ngSubmit)="onSubmit()">
<input type="text" formControlName="name">
<input type="email" formControlName="email">
<!-- add more form controls as needed -->
<button type="submit" [disabled]="myForm.invalid">Submit</button>
</form>
Display Validation Errors: आपण जे validation केले आहे त्याचा error msg आपल्या template मध्ये display करा. angular मध्ये formControl आपल्याला errors प्रॉपर्टी provide करते ज्या मध्ये validation error information असते.
example:
<div *ngIf="myForm.get('name').invalid && myForm.get('name').touched">
<div *ngIf="myForm.get('name').errors.required">Name is required</div>
</div>
<div *ngIf="myForm.get('email').invalid && myForm.get('email').touched">
<div *ngIf="myForm.get('email').errors.required">Email is required</div>
<div *ngIf="myForm.get('email').errors.email">Invalid email format</div>
</div>
Custom Validators: आपल्या requirement नुसार आपण custom validators सुद्धा add करू शकतो.
example:
function customValidator(control: FormControl): { [key: string]: any } | null {
const valid = // validation logic
return valid ? null : { custom: true };
}
// Then use it in form control definition
new FormControl('', customValidator);
Handling Form Submission: आपल्याला component मध्ये form submission handle करावे लागते.
example:
export class MyComponent {
onSubmit() {
if (this.myForm.valid) {
// Perform form submission logic
}
}
}
खाली आपण संपूर्ण example बघूया:
run this command to create new component: ng generate component ReactiveForm
reactive-form.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormControl, Validators } from '@angular/forms';
@Component({
selector: 'app-reactive-form',
templateUrl: './reactive-form.component.html',
styleUrls: ['./reactive-form.component.css']
})
export class ReactiveFormComponent implements OnInit {
myForm: FormGroup;
constructor() { }
ngOnInit(): void {
this.myForm = new FormGroup({
name: new FormControl('', Validators.required),
email: new FormControl('', [Validators.required, Validators.email]),
age: new FormControl('', [Validators.required, Validators.min(18)])
});
}
onSubmit() {
if (this.myForm.valid) {
console.log("Form submitted successfully!");
console.log(this.myForm.value);
} else {
console.log("Please fill in the required fields correctly.");
}
}
}
reactive-form.component.html
<form [formGroup]="myForm" (ngSubmit)="onSubmit()">
<div>
<label>Name:</label>
<input type="text" formControlName="name">
<div *ngIf="myForm.get('name').invalid && myForm.get('name').touched">
<div *ngIf="myForm.get('name').errors.required">Name is required</div>
</div>
</div>
<div>
<label>Email:</label>
<input type="email" formControlName="email">
<div *ngIf="myForm.get('email').invalid && myForm.get('email').touched">
<div *ngIf="myForm.get('email').errors.required">Email is required</div>
<div *ngIf="myForm.get('email').errors.email">Invalid email format</div>
</div>
</div>
<div>
<label>Age:</label>
<input type="number" formControlName="age">
<div *ngIf="myForm.get('age').invalid && myForm.get('age').touched">
<div *ngIf="myForm.get('age').errors.required">Age is required</div>
<div *ngIf="myForm.get('age').errors.min">Minimum age is 18</div>
</div>
</div>
<button type="submit" [disabled]="myForm.invalid">Submit</button>
</form>
reactive-form.component.css
label {
display: block;
margin-bottom: 5px;
}
input {
margin-bottom: 10px;
}
.error {
color: red;
}
3) What are observables in Angular? How are they used?
Angular मध्ये observable हे asynchronous data streams manage करण्याचा एक powerful मार्ग आहे. Observable हे RxJS library चा एक भाग आहे, ज्यामध्ये functions आणि classes चा सेट असतो ज्याचा वापर करून आपण reactive programming हि Observable वापरून करू शकतो.
Observable Angular मध्ये कसे use केले जाते ते बघूया:
1) Creation: आपण observable हे वेगवेगळ्या sources मार्फत करू शकतो जसे कि events, promises, arrays किंवा custom data sources.
2) Subscription: एकदा आपण observable create केले की आपल्याला त्या observable ला stream कडून emitted value ह्या receive करण्यासाठी subscribe करावे लागते. subscription मुळे तुम्हाला data stream मधील change वर react करता येते.
3) Consumption: subscription च्या आत observable कडून नवीन value emit झाल्यानंतर काय झाले पाहिजे ह्यासाठी आपण आपला code define करू शकतो. ह्यामध्ये UI update करणे, data processing करणे किंवा इतर काही action trigger करणे हे आपण करू शकतो.
4) Unsubscription: ज्यावेळेस Observable चे काम पूर्ण होते आणि त्याचा आता कुठे use होणार नसेल त्यावेळेस त्याला unsubscribe करणे खूप गरजेचे असते कारण तसे केले नाही तर memory leaks किंवा unnecessary processing होत राहील.
Observable हे Angular मध्ये commonly, HTTP requests handle करणे, user चे input manage करणे आणि real-time updates implement करणे ह्या प्रकारच्या task execute करण्यासाठी केला जातो.
Example:
import { Observable } from 'rxjs';
// Create an observable that emits values over time
const myObservable = new Observable<number>(observer => {
observer.next(1); // Emit the first value
observer.next(2); // Emit the second value
observer.next(3); // Emit the third value
setTimeout(() => {
observer.next(4); // Emit a fourth value after 1000ms
observer.complete(); // Complete the observable stream
}, 1000);
});
// Subscribe to the observable to receive emitted values
const subscription = myObservable.subscribe({
next: value => console.log(value), // Handle each emitted value
error: error => console.error(error), // Handle any errors
complete: () => console.log('Observable completed') // Handle completion of the observable
});
// Unsubscribe from the observable when no longer needed
subscription.unsubscribe();
4)Explain the role of RxJS in Angular applications (Observables and RxJS).
RxJS (Reactive Extensions for JavaScript) हि Observable चा वापर करून reactive programming करण्यासाठी एक powerful library आहे. RxJS चा वापर करून आपण asynchronous operations आणि data streams handle करू शकतो.
RxJS Angular सोबत कसे काम करते हे बघूया:
1) Observables and Streams: RxJS ने observable हि concept introduce केली आहे. Angular मध्ये Observable हे asynchronous operation handle करण्यासाठी वापरले जाते.
2) HTTP Requests: Angular चे HttpClient module हे HTTP request साठी observable return करते. ह्यामुळे developer RxJS operators वापरून data ला tranform, filter किंवा combine करू शकतो.
3) RxJS operators सोबत आपण Angular मध्ये Event Handling, State Management आणि real-time updates तसेच Error Handling आणि Retry logic ह्या activity करू शकतो.
थोडक्यात RxJS हे Angular मध्ये asynchronous programming ला simplify करते.
5)How do you make HTTP requests in Angular? Explain the HttpClient module (HTTP Client in marathi).
Angular मध्ये HTTP request आपण HttpClient module मार्फत करू शकतो. HttpClient module आपण अधिक डिटेल मध्ये बघूया
HttpClient module
Angular चे HttpClient module हे @angular/common/http ह्या package चा एक भाग आहे. हे module आपल्याला remote servers सोबत communicate करण्यासाठी HTTP request मार्फत एक powerful आणि straightforward API offer करते.
How we can use HttpClientModule in Angular?
1) Import HttpClientModule: सर्वप्रथम आपण आपल्या root module मध्ये म्हणजेच ‘AppModule’ मध्ये किंवा इतर feature module जिथे तुम्हाला HTTP services वापरायच्या आहे तिथे HttpClientModule हे इम्पोर्ट करून घ्या.
Example:
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
// components, directives, pipes
],
imports: [
BrowserModule,
HttpClientModule // Import HttpClientModule here
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
2) Inject HttpClient Service: आपल्या component किंवा service मध्ये HttpClient अगोदर import करून घ्या नंतर त्याला constructor मध्ये dependency Injection मार्फत inject करून घ्या.
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor(private http: HttpClient) { }
// Methods to make HTTP requests will be defined here
}
3) Making HTTP Requests: HttpClient आपल्याला हततप संबंधित GET, POST, PUT, DELETE, etc. ह्या काही method provide करते, जे आपल्याला HTTP responses चे observables return करते.
Example of GET request:
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
private apiUrl = 'https://api.example.com/data'; // Replace with your API URL
constructor(private http: HttpClient) { }
fetchData(): Observable<any> {
return this.http.get<any>(this.apiUrl);
}
}
4) Handling Responses: HTTP method जे observables return करते, ते आपल्याला subscribe,map ,catchError असे काही operator चा वापर करून response हाताळण्यासाठी मदत करते.
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-data',
templateUrl: './data.component.html',
styleUrls: ['./data.component.css']
})
export class DataComponent implements OnInit {
responseData: any;
constructor(private dataService: DataService) { }
ngOnInit(): void {
this.dataService.fetchData().subscribe(
(data) => {
this.responseData = data;
console.log(data);
},
(error) => {
console.error('Error fetching data: ', error);
}
);
}
}
6) What is the difference between HttpClient and Http?
Angular मध्ये HttpClient आणि Http हे server ला HTTP request करण्यासाठी वापरले जाते परंतु ह्या दोन्हींमध्ये थोडासा key difference आहे तो बघूया.
1) HttpClient:
- Module: HttpClient हे @angular/common/http चा part आहे आणि HttpClient हे Angular ४.३ मध्ये introduce केले गेले आहे.
- Feature: HttpClient हे HTTP requests साठी simplified API provide करते
- Interceptors: middleware logic handle करण्यासाठी interceptors ला support करते ज्यामुळे आपण adding headers किंवा globally error handling सारख्या गोष्टी करू शकतो.
- Immutability: HttpClient चे insatances हे immutable आहेत म्हणजेच प्रत्येक नवीन request ला नवीन instance तयार केला जातो, ह्या मध्ये existing instance ला mutating केले जात नाही.
- RxJS: HttpClient हे request पासून Observable object रिटर्न करते. ज्यापासून आपण RxJS चे ऑपरेटर वापरून asynchronous data handle करू शकतो.
2) Http:
- Module: HttpClient introduce झाल्यानंतर Angular ४.३ पासून Http हे Deprecated झाले आहे परंतु @angular/http चा part आहे.
- Features: HttpClient मध्ये request handle करणे Http पेक्षा सोपे आहे कारण Http मध्ये request हॅन्डल करण्यासाठी कोड कॉम्प्लेक्स आहे.
- Promises: request पासून Promise object return केला जातो
- Mutable Instance: Http instances हे mutable आहेत
- Interceptors: Http हे interceptors ला support करत नाही