Lets Understand Unit Test for Angular in marathi (Unit Testing in Angular in marathi)
1) What testing frameworks are commonly used in Angular? (Testing in Angular in marathi)
Angular development मध्ये angular application test करण्यासाठी काही testing framework commonly वापरले जातात. testing framework वापरून unit testing, integration testing, and end-to-end (e2e) testing हे cover केले जाते.
काही main testing framework बघूया:
1) Karma: Karma हे Angular application चे default test runner म्हणून काम करते. Karma framework हे tests Jasmin आणि Mocha चा वापर करून वेगवेगळ्या browsers मध्ये execute करते आणि test environment provide करते. Karma हे Angular CLI सोबत unit tests run करण्यासाठी well-integrated आहे.
2) Jasmine: Jasmine हे behavior-driven development (BDD) फ्रेमवर्क असून ते JavaScript code test करण्यासाठी वापरले जाते. हे Angular मध्ये unit tests लिहण्यासाठी Angular ची default choice आहे.
3) Protractor: Protractor हे एक end-to-end (e2e) testing framework आहे जे specifically Angular applications साठी design केले आहे. हे framework आपल्या ऍप्लिकेशनच्या tests ह्या real browser मध्ये run करते.
4) Jest: Jest framework हे त्याच्या speed आणि developer experience मुळे चांगलेच लोकप्रिय आहे. Jest framework हे unit testing आणि snapshot testing ह्या दोन्ही testing साठी Angular application मध्ये वापरले जाते.
5) Cypress: Cypress framework हे Angular application च्या end-to-end testing साठी लोकप्रिय फ्रेमवर्क आहे. हे browser मध्ये operate करता येते आणि आपल्याला tests अधिक interactive आणि real-time manner ने write करता येतात.
2) How do you write unit tests and end-to-end tests in Angular?
Unit Tests:
1.Set Up Angular Testing Environment:
Angular CLI हे karma आणि Jasmine चा वापर करून default testing environment set up करते. तुमच्या प्रोजेक्ट मध्ये हे default configuration आहे कि नाही हे चेक करा.
- Write Unit Tests with Jasmine:
.spec.ts extension असलेली फाईल तुमच्या component मध्ये आहे का हे चेक करा नसेल तर ती create करा.
Jasmine syntax (describe, it, expect, etc) वापरून टेस्ट्स write करा
- TestBed Configuration:
TestBed.configureTestingModule चा वापर करून तुम्हाला जे component किंवा service test करायची आहे त्यासाठी testing module तयार करा. - Inject Dependencies:
Angular dependency injection system वापरून तुम्हाला जे component test करायचे आहे, त्यासाठी लागणारी service inject करून घ्या. - Mock Dependencies:
Jasmine चे spies जसे कि jasmine.createSpy or spyOn ह्यांचा वापर करून mock dependencies किंवा behavior control करा. - Trigger Change Detection:
fixture.detectChanges() चा वापर करून Angular component मधील change detect करा आणि त्याचे rendered output test करा
Example
import { TestBed, ComponentFixture } from '@angular/core/testing';
import { MyComponent } from './my.component';
import { DataService } from './data.service';
describe('MyComponent', () => {
let component: MyComponent;
let fixture: ComponentFixture<MyComponent>;
let dataServiceSpy: jasmine.SpyObj<DataService>;
beforeEach(() => {
const spy = jasmine.createSpyObj('DataService', ['getData']);
TestBed.configureTestingModule({
declarations: [ MyComponent ],
providers: [
{ provide: DataService, useValue: spy }
]
});
fixture = TestBed.createComponent(MyComponent);
component = fixture.componentInstance;
dataServiceSpy = TestBed.inject(DataService) as jasmine.SpyObj<DataService>;
});
it('should create', () => {
expect(component).toBeTruthy();
});
it('should fetch data on ngOnInit', () => {
const testData = 'Test Data';
dataServiceSpy.getData.and.returnValue(testData);
fixture.detectChanges(); // Trigger ngOnInit
expect(component.data).toEqual(testData);
});
});
End-to-End (e2e) Tests:
- Set Up Angular e2e Testing Environment:
e2e testing साठी Protractor or Cypress framework चा वापर करा - Write e2e Tests:
.e2e-spec.ts ह्या extension ने file create करा व Jasmine’s syntax हे Protractor किंवा Cypress commands सोबत वापरून tests write करा - Describe Test Scenarios:
‘describe’ आणि ‘it’ blocks वापरून test scenario define करा वexpect
statement वापरून assertion define करा - Interact with the Application:
Protractor or Cypress commands (element, by, click, type, etc.) वापरून page वरील elements सोबत interaction करू शकता - Handle Asynchronous Operations:
Protractor किंवा Cypress commands ह्या async and await सोबत वापरून asynchronous operations handle करा.
Example:
describe('App', () => {
beforeEach(() => {
browser.get('/');
});
it('should display welcome message', () => {
expect(element(by.css('app-root h1')).getText()).toEqual('Welcome to my-app!');
});
it('should navigate to about page', () => {
element(by.css('a[routerLink="/about"]')).click();
expect(browser.getCurrentUrl()).toMatch(/\/about$/);
expect(element(by.css('h2')).getText()).toEqual('About Us');
});
});
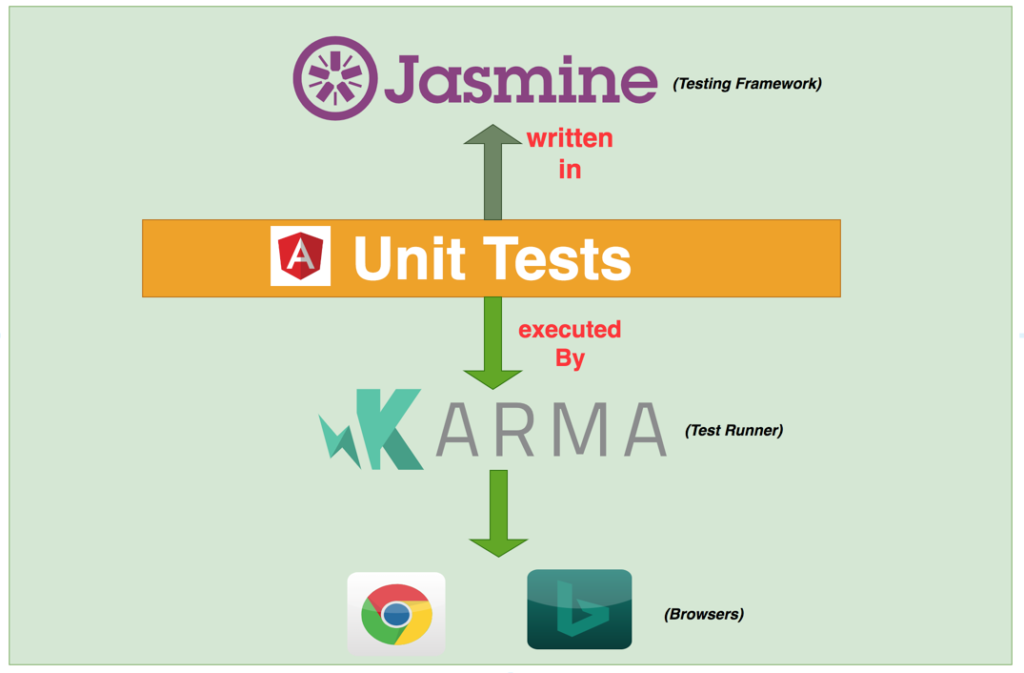
3) Explain lazy loading in Angular. Why is it beneficial? (Angular Interview questions on advanced topics)
Angular मध्ये Lazy loading म्हणजे फक्त required module ज्या वेळेस गरज आहे त्यावेळेस लोड करण्याची technique आहे. Lazy loading ह्या Angular च्या feature मुळे ऍप्लिकेशनचा सुरवातीचा loading time कमी होतो त्यामुळे ऍप्लिकेशनचा performance काही प्रमाणात सुधारण्यास मदत होते.
Lazy loading कसे beneficial आहे ते बघूया:
1) Faster Initial Load: फक्त जे आवश्यक module आहेत तेवढेच गरजेनुसार load करून initial load टाइम कमी करून ऍप्लिकेशन फास्ट लोड होऊ शकते.
2) Improved Performance and Reduced Initial Payload: larger application साठी सगळं काही एकाच वेळेस लोड केल्यावर त्याचा सरळ performance वरती impact होतो. त्यासाठी जर आपण smaller initial bundles म्हणजेच data transfer ऍप्लिकेशन लोड होत असताना जर कमी केला तर initial load time कमी होऊन ऍप्लिकेशनचा performance वाढतो.
3) Optimized Use of Resources: Lazy loading feature मुळे Angular ला resources हे अधिक efficiently allocate करण्यास मदत होते कारण memory सारखे resource हे फक्त जेवढे modules loaded आहेत त्यांनाच allocate केले जाते. त्यामुळे इतर modules जे लोड झालेले नाहीत त्यांना resource allocate नाही करावा लागत.
lazy loading हे router configure करून achieve केले जाते, routing मध्ये loadChildren हे property वापरून modules हे asynchronously load केले जातात. loadChildren ह्या प्रॉपर्टी मध्ये module चा path आणि त्या module चे class name आपल्याला define करावे लागते.
Example:
const routes: Routes = [
{ path: 'dashboard', loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule) },
{ path: 'profile', loadChildren: () => import('./profile/profile.module').then(m => m.ProfileModule) },
// other routes
];
4) What are Angular decorators? Provide examples.
Angular मध्ये decorators हे थोडक्यात function आहे जे classes आणि class members ला metadata सोबत अधिक माहिती (explanation) देतात. Angular मधील Components, directives, services कशे process झाले पाहिजे किंवा कशे behave केले पाहिजे ह्याबद्दल माहिती किंवा configuration हे decorators provide करते.
Lets see some commonly used decorators in Angular along with examples:
1) @Component: @Component decorator हे class ला Angular component म्हणून mark करण्यासाठी व component बद्दल असलेला metadata provide करण्यासाठी वापरले जाते, जसे की selector, template किंवा templateUrl, styles किंवा styleUrls, इत्यादी.
Example:
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent {
// Component logic goes here
}
2) @Directive: @Directive decorator हे class ला Angular Directive म्हणून मार्क करण्यासाठी वापरले जाते आणि directive च्या behavior बद्दल metadata provide करण्यासाठी वापरले जाते.
Example:
import { Directive, ElementRef, HostListener } from '@angular/core';
@Directive({
selector: '[appHighlight]'
})
export class HighlightDirective {
constructor(private el: ElementRef) { }
@HostListener('mouseenter') onMouseEnter() {
this.highlight('yellow');
}
@HostListener('mouseleave') onMouseLeave() {
this.highlight(null);
}
private highlight(color: string | null) {
this.el.nativeElement.style.backgroundColor = color;
}
}
3) @Injectable: @Injectable decorator हे class मध्ये dependencies आहेत आणि त्या constructor मध्ये inject कराव्या लागू शकतात जेव्हा dependency injector त्या क्लासचा instance create करेल तेव्हा, ह्यासाठी @injectable डेकोरेटर वापरला जातो.
Example:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class DataService {
// Service logic goes here
}
4) @Input and @Output:
हे decorators component किंवा directives च्या input किंवा output properties define करण्यासाठी वापरले जाते. Parent component to child component मध्ये ज्यावेळेस data sharing करायची असते त्यावेळेस @Input() डेकोरेटर वापरले जाते.
तसेच ज्यावेळेस child component ते parent component मध्ये data sharing करायची असते त्यावेळेस @Output() हे डेकोरेटर वापरले जाते.
Example:
@Input Example :
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: `<p>{{ message }}</p>`
})
export class ChildComponent {
@Input() message: string;
}
@Output example:
import { Component, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-child',
template: `<button (click)="sendMessage()">Send Message</button>`
})
export class ChildComponent {
@Output() messageEvent = new EventEmitter<string>();
sendMessage() {
this.messageEvent.emit('Hello from child');
}
}